Machine Learning Projects With Source Code
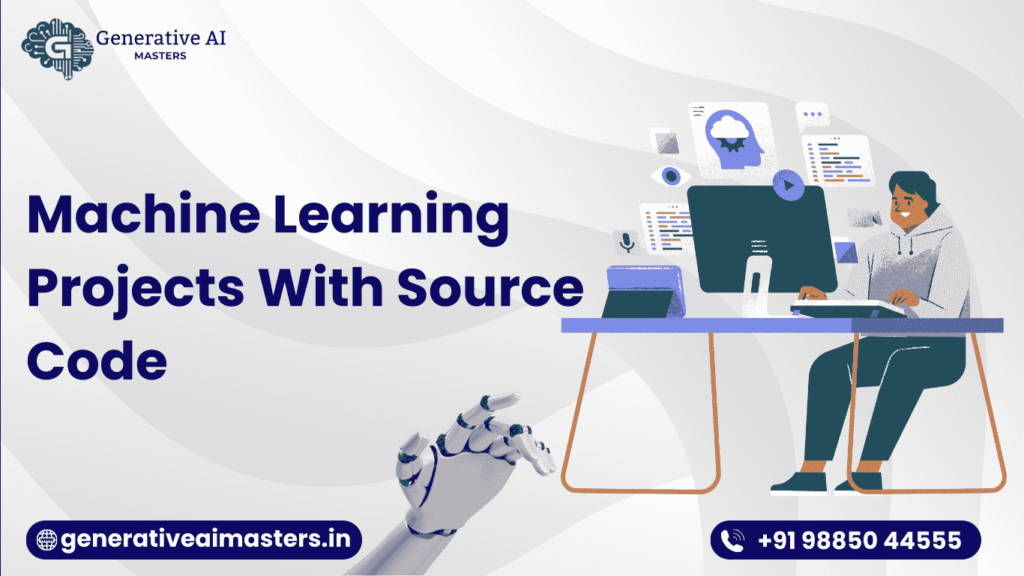
Machine Learning Projects With Source Code
House Price Prediction
House price prediction is a common machine learning problem that involves using various features (such as the area, number of rooms, location, etc.) of houses to predict their prices. This task typically uses regression models, as the output is a continuous value (the price). With the help of historical house data, a machine learning model can be trained to predict prices for new houses.
Steps to Build the Project:
1. Set Up the Development Environment
Install necessary libraries like Pandas, NumPy, Scikit-learn, Matplotlib, and Seaborn for data manipulation and model building.
2. Data Collection
3. Data Preprocessing
- Clean the dataset by handling missing values, outliers, and duplicate records.
- Encode categorical features like the house type and location using one-hot encoding or label encoding.
4. Feature Engineering
- Extract relevant features such as area, number of rooms, location, year built, and other factors that might affect house prices.
- Normalize or standardize numerical features where necessary.
5. Model Selection
- Choose appropriate regression algorithms such as Linear Regression, Random Forest Regressor, Gradient Boosting Regressor, or XGBoost for the task.
6. Model Training
- Split the data into training and testing sets, then train the model using the training data.
- Fine-tune hyperparameters using GridSearchCV or RandomizedSearchCV.
7. Model Evaluation
- Evaluate the model’s performance using metrics like Mean Absolute Error (MAE), Mean Squared Error (MSE), and R-squared.
8. Prediction
- Use the trained model to predict house prices for new data. Visualize the results and compare the predicted prices with the actual prices.
Real-Time Applications
1. Real Estate Price Estimation
- Real estate companies can use such models to estimate the price of properties in the market based on given features, enabling better pricing strategies.
2. Investment Decision Making
- Investors can use the model to forecast future house prices and make informed decisions about buying or selling properties.
3. Property Valuation
- Banks and financial institutions can use the model for property valuation during mortgage loan approvals.
Source Code:
YouTube Tutorial:
Iris Flower Classification
Learning Outcomes:
1. Understanding Machine Learning Concepts
2. Data Manipulation with Python
3. Exploratory Data Analysis (EDA)
4. Model Implementation
5. Model Evaluation
6. Model Training
Steps to Execute the Project:
1. Set Up the Environment
Pandas, NumPy, Matplotlib, Seaborn, and Scikit-learn.
2. Data Collection
3. Data Preprocessing
- Handle missing values (if any).
- Encode categorical variables.
- Normalize or standardize the data if required.
4. Exploratory Data Analysis (EDA)
- Plot histograms, scatter plots, and pair plots to understand data distributions and relationships.
- Identify any patterns or correlations in the data.
5. Model Training
- Split the data into training and testing sets.
- Train various classification models (e.g., KNN, Decision Tree, SVM) on the training data.
- Tune hyperparameters to optimize model performance.
6. Model Evaluation
- Evaluate models on the test set using accuracy, precision, recall, and F1-score.
- Compare model performances to select the best one.
7. Model Deployment
- Deploy the selected model using frameworks like Flask or Django to create a web application for user interaction.
Real-Time Applications:
1. Educational Purposes
- Serves as an introductory project for learning machine learning concepts and techniques.
2. Botanical Research
- Assists in automating the classification of iris species based on morphological features.
3. Pattern Recognition Systems
- Provides foundational understanding applicable to more complex classification tasks in various domains.
Source Code:
YouTube Tutorial
Handwritten Digit Recognition
Learning Outcomes:
1. Introduction to Deep Learning
- Understand the fundamentals of neural networks and deep learning models used in image recognition.
2. Data Preprocessing
- Learn to load and preprocess image data, including normalization and reshaping using TensorFlow and Keras.
3. Model Building
- Build and train neural networks for digit recognition using Convolutional Neural Networks (CNNs).
4. Model Evaluation
- Evaluate model performance using metrics like accuracy and confusion matrix to determine the model’s effectiveness.
5. Model Optimization
- Improve model performance by tuning hyperparameters and adding layers or dropout for regularization.
6. Deployment
- Deploy the trained model for real-time digit prediction using TensorFlow Serving or other deployment methods.
Steps to Execute the Project:
1. Set Up the Development Environment
2. Load the MNIST Dataset
3. Preprocess the Data
- Normalize the pixel values (scaling them to a range between 0 and 1).
- Split the dataset into training and testing sets.
4. Build the Neural Network
- Create a Convolutional Neural Network (CNN) with layers like Conv2D, MaxPooling2D, Flatten, and Dense.
- Use activation functions like ReLU for hidden layers and softmax for the output layer to classify digits.
5. Train the Model
6. Evaluate the Model
7. Optimize the Model
8. Deployment
Deploy the trained model for use in real-time applications, such as digit recognition on mobile apps or web platforms.
Real-Time Applications:
1. Automated Postal Sorting
2. Handwritten Data Entry
3. Optical Character Recognition (OCR)
Source Code:
YouTube Tutorial:
Spam Email Detection
Learning Outcomes:
1. Introduction to NLP
2. Text Preprocessing
3. Feature Extraction
4. Model Building
5. Model Evaluation
6. Model Optimization
7. Deployment
Steps to Execute the Project:
1. Set Up the Development Environment
2. Load the Dataset
3. Preprocess the Text Data
- Clean the email text by removing special characters, stop words, and non-alphabetical characters.
- Tokenize the text and apply stemming or lemmatization to reduce words to their base form.
4. Feature Extraction
5. Train a Classification Model
- Split the dataset into training and testing sets.
- Train models like Naive Bayes, Logistic Regression, or SVM on the training set.
6. Evaluate the Model
7. Optimize the Model
8. Deploy the Model
Real-Time Applications:
1. Email Filtering
2. Customer Support
3. Marketing
Source Code:
YouTube Tutorial:
Stock Price Prediction
Learning Outcomes:
1. Introduction to Time-Series Analysis
2. Data Preprocessing
3. Exploratory Data Analysis (EDA)
4. Time-Series Forecasting Techniques
5. Model Evaluation
6. Model Optimization
7. Deployment
Steps to Execute the Project:
1. Set Up the Development Environment
2. Load Historical Stock Data:
3. Preprocess the Data
- Clean the data by handling missing values, normalizing the data, and transforming it into a time-series format.
- Split the data into training and testing sets.
4. Visualize the Data
5. Train a Time-Series Model
- Start with simpler models like ARIMA for forecasting.
- For more complex predictions, use deep learning models like LSTM to capture long-term dependencies in the data.
6. Evaluate the Model
7. Optimize the Model
8. Deployment
Real-Time Applications:
1. Stock Market Prediction
2. Trading Algorithms
3. Portfolio Management
Source Code:
YouTube Tutorial:
Breast Cancer Detection
Learning Outcomes:
1. Introduction to Classification
Learn how to apply machine learning algorithms to binary classification tasks (malignant vs. benign).
2. Data Preprocessing
3. Feature Engineering
4. Model Building
5. Model Evaluation
6. Model Optimization
7. Deployment
Steps to Execute the Project:
1. Set Up the Development Environment
2. Load the Dataset
3. Preprocess the Data
4. Train the Model
5. Evaluate the Model
6. Optimize the Model
7. Deploy the Model
Real-Time Applications:
1. Medical Diagnosis
2. Automated Screening
3. Healthcare Decision Support
YouTube Tutorial:
Customer Churn Prediction
Learning Outcomes:
1. Customer Churn Analysis
2. Data Preprocessing
3. Feature Engineering
4. Model Building
5. Model Evaluation
6. Model Optimization
7. Deployment
Steps to Execute the Project:
1. Set Up the Development Environment:
2. Load Customer Data
3. Preprocess the Data
4. Train the Model
5. Evaluate the Model
6. Optimize the Model
7. Deploy the Model
Real-Time Applications:
1. Customer Retention
2. Business Strategy
3. Resource Allocation
YouTube Tutorial:
Sentiment Analysis
Learning Outcomes:
1. Introduction to Sentiment Analysis
2. Data Preprocessing
3. Feature Engineering
4. Model Building
5. Model Evaluation
6. Model Optimization
7. Deployment
Steps to Execute the Project:
1. Set Up the Development Environment
2. Load Review Data
3. Preprocess the Data
- Clean the text data by removing punctuation, converting to lowercase, and removing stopwords.
- Transform the text into numerical features using TF-IDF or word embeddings.
4. Train the Model
5. Evaluate the Model
6. Optimize the Model
7. Deploy the Model
Real-Time Applications:
1. Customer Feedback Analysis:
2. Brand Monitoring
3. Market Research
YouTube Tutorial:
Movie Recommendation System
1. Content-Based Filtering
- Recommends movies similar to what the user has already liked.
- Uses movie metadata like genre, director, cast, etc.
2. Collaborative Filtering
- Recommends movies based on similarities between users or items.
- Leverages user-item interaction data like ratings or watch history.
3. Hybrid Approach
Steps to Build:
1. Set Up the Environment
2. Data Preparation
- Use a dataset like the MovieLens dataset available on Kaggle.
- Clean and preprocess data to handle missing or inconsistent values.
3. Model Implementation
- Content-Based: Use cosine similarity or TF-IDF to recommend movies based on metadata.
- Collaborative Filtering: Apply algorithms like Singular Value Decomposition (SVD).
- Hybrid: Combine both approaches for better results.
4. Model Evaluation
Use metrics like Precision, Recall, and RMSE (Root Mean Squared Error) for collaborative filtering.
5. Deployment
Real Time Applications:
- OTT Platforms: Netflix and Hulu use similar systems to suggest movies.
- E-commerce Platforms: Recommending products based on customer behavior.
- Educational Platforms: Suggesting courses based on learning history.
YouTube Tutorial:
Face Detection Using OpenCV
This project involves detecting faces in images or videos using the OpenCV library. OpenCV’s pre-trained Haar cascades or Deep Learning-based methods can be used for accurate face detection.
Steps to Build the Project:
1. Set Up the Environment
Install Python and OpenCV library using pip install opencv-python.
2. Load the Haar Cascade Classifier
3. Read Image/Video
Use OpenCV's cv2.imread() for images and cv2.VideoCapture() for video streams.
4. Apply Face Detection:
Detect faces using cv2.CascadeClassifier.detectMultiScale() for Haar cascades or a deep learning model such as DNN-based face detectors.
5. Display Detected Faces
Draw bounding boxes around detected faces using cv2.rectangle().
6. Real-Time Detection (Optional)
Real-Time Applications:
1. Security Systems
2. Attendance Systems
3. Photo Applications
YouTube Tutorial:
Text Summarization
This project involves developing a system to automatically summarize long documents or articles using Natural Language Processing (NLP) techniques. Text summarization can be either:
1. Extractive Summarization
2. Abstractive Summarization
Steps to Build the Project:
1. Set Up the Environment
2. Load the Text Data
3. Preprocess the Text
4. Apply Summarization Technique
- Extractive Approach: Use algorithms like TextRank to select key sentences based on importance.
- Abstractive Approach: Use pre-trained transformer models like BERT, GPT, or T5 for abstractive summarization.
5. Evaluate the Summarization
6. Deploy the Summarizer
Real-Time Applications:
1. Content Creation
2. News Aggregators
3. Customer Support
YouTube Tutorial:
Object Detection
Object Detection
1. Set Up the Environment
2. Load a Pre-trained Model
3. Prepare the Dataset (Optional)
4. Run the Model:
5. Visualize Results
6. Optimize the Model
7. Deploy the Model
Real-Time Applications:
1. Security Systems
2. Autonomous Vehicles
3. Retail and Inventory
4. Healthcare
YouTube Tutorial:
Credit Card Fraud Detection
This project involves detecting fraudulent transactions from financial data using machine learning techniques. The goal is to classify transactions as either legitimate or fraudulent based on various features like transaction amount, merchant information, and user behavior.
Steps to Build the Project:
1. Set Up the Environment
2. Load and Preprocess Data
- Use datasets such as the Kaggle Credit Card Fraud Detection Dataset.
- Clean the data, handle missing values, and perform feature engineering to extract useful information.
3. Feature Selection/Engineering
4. Model Building
5. Visualize Results
6. Handle Imbalanced Data
7. Deploy the Model
Real-Time Applications:
1. Security Systems
2. E-commerce Platforms
3. Insurance Industry
YouTube Tutorial:
Chatbot Using Rasa
Steps to Build the Project:
1. Set Up the Environment
2. Load and Preprocess Data
3. Define NLU Data
4. Define Stories and Rules
5. Visualize Results
6. Run the Action Server (if needed)
7. Test and Improve the Bot
8. Deploy the Chatbot (Optional)
Real-Time Applications:
1. Customer Support
2. Sales and Marketing
3. E-commerce
4. Healthcare
YouTube Tutorial:
Style Transfer with Neural Networks
Steps to Build the Project:
1. Set Up the Environment
- Install required libraries such as TensorFlow or PyTorch, and Keras for building and training neural networks.
- You can also use pre-trained models like VGG16 or VGG19 for the style and content feature extraction.
2. Load the Images
3. Pre-process the Images
4. Extract Features Using Pre-trained Models
5. Define Loss Functions
- Content Loss: Measures how much the content of the generated image differs from the original content image.
- Style Loss: Measures how much the generated image’s style differs from the target style image.
- Total Loss: A weighted combination of content and style loss to optimize during training.
6. Optimize the Image
7. Output the Final Image
Real-Time Applications:
1. Digital Art
2. Video Game Design
3. E-commerce
4. Creative Projects
YouTube Tutorial:
Image Caption Generator
Steps to Build the Project:
1. Set Up the Environment
2. Load and Preprocess the Dataset
- Use datasets like Microsoft COCO or Flickr8k for image captioning tasks. These datasets consist of images paired with human-generated captions.
- Preprocess the images by resizing and normalizing them, and the captions by tokenizing and padding.
3. Extract Image Features
4. Extract Features Using Pre-trained Models
- Use a combination of a CNN for image feature extraction and an RNN or LSTM for generating sequences of words based on the extracted features.
- The LSTM will generate word sequences that form coherent captions for the given images.
5. Train the Model
6. Optimize the Image
7. Output the Final Image
Real-Time Applications:
1. igital Art
2. Video Game Design
3. E-commerce
4. Image Search Engines
YouTube Tutorial:
Time-Series Forecasting
Steps to Build the Project:
1. Set Up the Environment
2. Load and Preprocess the Data
3. Visualize the Data
4.Stationarity Testing
5. Choose and Build a Model
- For traditional methods, you can use ARIMA (Auto-Regressive Integrated Moving Average) or SARIMA (seasonal ARIMA).
- Alternatively, deep learning methods such as LSTM can be used for more complex time-series datasets with long-term dependencies.
6. Train the Model
7. Evaluate the Model
8. Forecast Future Values
9. Optimize and Improve the Model
Real-Time Applications:
1. Sales Forecasting
2. Weather Forecasting
3. Financial Market Forecasting
4. Demand Forecasting
YouTube Tutorial:
Fake News Detection
Steps to Build the Project:
1. Set Up the Environment
2. Load and Preprocess the Data
- Load a dataset containing labeled news articles (e.g., Fake News Dataset from Kaggle or LIAR dataset).
- Clean the text data by removing stopwords, punctuation, and performing tokenization and stemming/lemmatization.
3. Feature Extraction
4. Build the Classification Model
- Choose an algorithm for classification such as Naive Bayes, Logistic Regression, or Random Forest.
- For more advanced models, you can use Deep Learning with LSTM or BERT to analyze the textual data.
5. Train the Model
6. Evaluate the Model
7. Improve the Model
8. Deploy the Model
Real-Time Applications:
1. Social Media Monitoring
2. News Agencies
3. Government and Regulatory Bodies
4. Fact-Checking Tools
YouTube Tutorial:
Speech Emotion Recognition
Speech Emotion Recognition (SER) involves detecting emotions such as happiness, sadness, anger, or fear from speech or audio data. This project uses machine learning and deep learning techniques to analyze speech signals and classify the emotion expressed in the voice. The typical workflow includes feature extraction, model training, and evaluation.
Steps to Build the Project:
1. Set Up the Environment
2. Load and Preprocess the Audio Data
- Use a dataset such as the RAVDESS dataset or TESS dataset, which contains speech data labeled with emotions.
- Preprocess the audio files by extracting relevant features, such as MFCCs (Mel Frequency Cepstral Coefficients), Chroma, Spectral Contrast, or Zero-Crossing Rate.
3. Feature Extraction
4. Build the Model
- Use algorithms like Random Forest, SVM, or Neural Networks (e.g., CNN or LSTM) to train the model.
- For deep learning models, Convolutional Neural Networks (CNN) can be used to capture local features from spectrograms of the audio.
5. Train the Model
Split the dataset into training and testing sets, then train the model using the extracted features.
6. Evaluate the Model
Use evaluation metrics such as Accuracy, Precision, Recall, and F1-Score to evaluate how well the model is detecting emotions from the speech data.
7. Improve the Model
8. Deploy the Model
Real-Time Applications:
1. Customer Service
2. Healthcare
3. Government and Regulatory Bodies
4. Security Systems
YouTube Tutorial:
Reinforcement Learning: CartPole Balancing
The CartPole Balancing problem is a classic reinforcement learning task where the objective is to balance a pole on a moving cart. The agent receives feedback in the form of rewards based on its actions (moving left or right), and the goal is to train the agent to maintain the pole in a balanced position for as long as possible.
Steps to Build the Project:
1. Set Up the Environment
2. Understand the Environment:
3. Feature Extraction
4. Set Up the Learning Algorithm
5. Training the Agent
6. Evaluate the Performance
7.Improve the Agent
8. Deploy the Model
Real-Time Applications:
1. Robotics
2.Autonomous Vehicles
3. Control Systems:
YouTube Tutorial:
Faq's
- Depending on the problem (classification, regression, clustering, etc.), common algorithms like Linear Regression, Decision Trees, Random Forests, SVMs, K-Means, Neural Networks, or XGBoost may be used.
You should choose a dataset based on the problem you are trying to solve. Datasets should have enough features and data points to train the model effectively. Websites like Kaggle, UCI Machine Learning Repository, and Google Dataset Search are good sources for datasets.
- Data preprocessing is crucial for cleaning the data, handling missing values, scaling features, encoding categorical variables, and splitting the dataset into training and testing subsets to ensure accurate and efficient model training.
- Evaluation metrics like accuracy, precision, recall, F1-score, RMSE, and AUC help assess the performance of the machine learning model and guide improvements to make it more reliable and efficient.
- Techniques like cross-validation, regularization (Lasso, Ridge), pruning decision trees, and early stopping in neural networks are used to prevent overfitting. Reducing the complexity of the model can also help.
- Feature engineering involves creating new features from raw data, removing irrelevant ones, or transforming existing features to improve model performance. It can significantly enhance a model’s ability to generalize.
- Grid Search and Random Search are two common methods to tune hyperparameters. More advanced techniques like Bayesian Optimization or Genetic Algorithms can also be used for hyperparameter tuning.
Popular machine learning frameworks and libraries include Scikit-learn, TensorFlow, Keras, PyTorch, XGBoost, LightGBM, and Fastai.
- You can deploy a model by converting it into an API using frameworks like Flask, FastAPI, or Django, and deploying it to cloud platforms like AWS, Google Cloud, or Azure. For real-time predictions, you can also use Docker to containerize the model.
- If your model is underperforming, you can try the following:
- Collect more data or use more relevant features.
- Fine-tune the model by adjusting hyperparameters.
- Apply more advanced algorithms or try ensemble methods.
- Check for class imbalance and address it using techniques like SMOTE or undersampling.
Tools like SHAP (SHapley Additive exPlanations) and LIME (Local Interpretable Model-agnostic Explanations) can help interpret black-box models, providing insights into which features influence model predictions.
- Common mistakes include:
- Using low-quality or biased data.
- Not splitting the dataset correctly (train-test split).
- Ignoring cross-validation and hyperparameter tuning.
- Overfitting the model without checking its generalization performance.
- Popular repositories for machine learning projects with source code include:
- GitHub – Search for machine learning projects tagged with “machine-learning” or “deep-learning”.
- Kaggle – Provides datasets and example notebooks for a variety of machine learning problems.
- Awesome Machine Learning – A curated list of machine learning frameworks, tools, and resources on GitHub.
The success of a project can be measured by evaluating the model’s performance using appropriate metrics, ensuring it meets the project’s objectives, and considering business impact if applicable.
Unsupervised learning algorithms like K-means clustering, DBSCAN, and Autoencoders are used when the data does not have labeled outputs, such as in anomaly detection, clustering, or dimensionality reduction tasks.
Want to learn more about Generative AI ?
Join our Generative AI Masters Training Center to gain in-depth knowledge and hands-on experience in generative AI. Learn directly from industry experts through real-time projects and interactive sessions.